Only
Return whitelisted properties of an object.
Only will only return the properties of an object that we are intrested in.
A simple and an effective module from Tj Holowaychuk .
Install it: npm install only
Sample usage:
Say we have a response from a mongo query which looks like:
1
2
3
4
5
6
| var obj = {
name: 'tobi',
last: 'holowaychuk',
email: '[email protected]',
_id: '12345'
};
|
Out of which we are intrested on only name
and email
, so all we need to do is:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
| let only = require('only');
var obj = {
name: 'tobi',
last: 'holowaychuk',
email: '[email protected]',
_id: '12345'
};
console.log(only(obj,'name email'));
// ^ Would log { name: 'tobi', email: '[email protected]' }
console.log(only(obj,'name foo bar baz'));
// Smart enough to omit undefineds and logs { name: 'tobi' }
|
The cool part being, the entire module is just ten lines and it reads:
1
2
3
4
5
6
7
8
9
| module.exports = function(obj, keys){
obj = obj || {};
if ('string' == typeof keys) keys = keys.split(/ +/);
return keys.reduce(function(ret, key){
if (null == obj[key]) return ret;
ret[key] = obj[key];
return ret;
}, {});
};
|
GIF FTW!
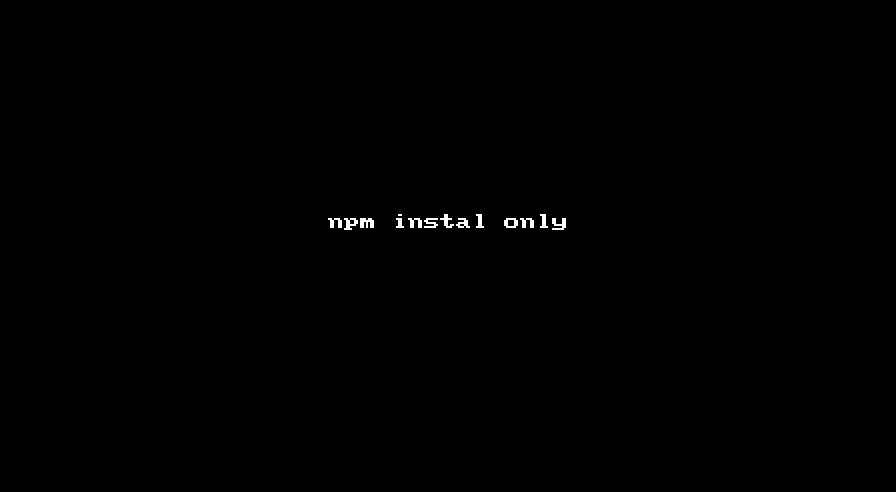