url-pattern
easier than regex string matching for urls, domains, filepaths and other strings.
url-pattern
can capture named parts of strings and conveniently returns them as objects.
Also does the reverse and generates strings given a pattern and such an object.
install: npm install url-pattern
simple match example:
1
2
3
4
5
6
7
8
9
| var UrlPattern = require('url-pattern');
var pattern = new UrlPattern('/api/users/:id');
pattern.match('/api/users/10');
{id: '10'}
pattern.match('/api/products/5');
null
|
complex match example showing off escaping, wildcards and optional segments:
1
2
3
4
5
6
7
8
9
| var pattern = new UrlPattern('(http(s)\\://)(:subdomain.):domain.:tld(/*)')
pattern.match('google.de'); // {domain: 'google', tld: 'de'}
pattern.match('https://www.google.com'); // {subdomain: 'www', domain: 'google', tld: 'com'}
pattern.match('http://mail.google.com/mail'); // {subdomain: 'mail', domain: 'google', tld: 'com', _: 'mail'}
pattern.match('google') ; // null
|
stringify example:
1
2
3
4
5
| var pattern = new UrlPattern('/api/users(/:id)');
pattern.stringify() // '/api/users'
pattern.stringify({id: 10}) //' /api/users/10'
|
GIF FTW!
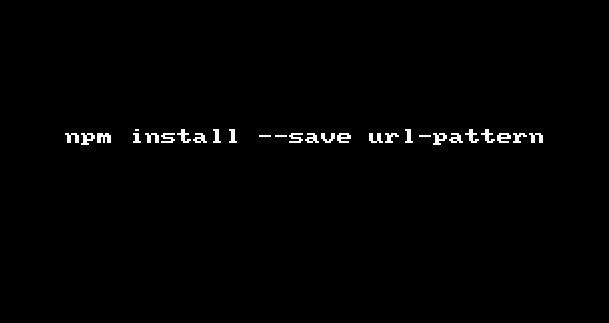